Private Package Registry on git
Prerequisites
- Node.js installed
- GitHub account
- GitHub Personal Access Token with repo and packages:write scopes
Create a Typescript
mkdir my-typescript-library
cd my-typescript-library
npm init -y
npm install -D typescript @types/node ts-node
npx tsc --init
Create code
Create src/index.ts:
export function greet(name: string): string {
return `Hello, ${name}!`;
}
Configure your package.json and tsconfig
{
"name": "@your-github-username/my-typescript-library",
"version": "1.0.0",
"description": "A sample TypeScript library",
"main": "dist/index.js",
"types": "dist/index.d.ts",
"scripts": {
"build": "tsc",
"prepare": "npm run build"
},
"publishConfig": {
"registry": "https://npm.pkg.github.com/"
},
"repository": {
"type": "git",
"url": "https://github.com/your-github-username/my-typescript-library.git"
},
"keywords": ["typescript", "library"],
"author": "Your Name",
"license": "MIT"
}
{
"compilerOptions": {
"target": "es6",
"module": "commonjs",
"declaration": true,
"outDir": "./dist",
"strict": true
},
"include": ["src"],
"exclude": ["node_modules"]
}
Authenticate with github packages
Generate a Personal Access Token (Classic)
- Go to GitHub Settings > Developer Settings > Personal Access Tokens
- Create a token with repo and packages:write scopes
- Login to GitHub Packages
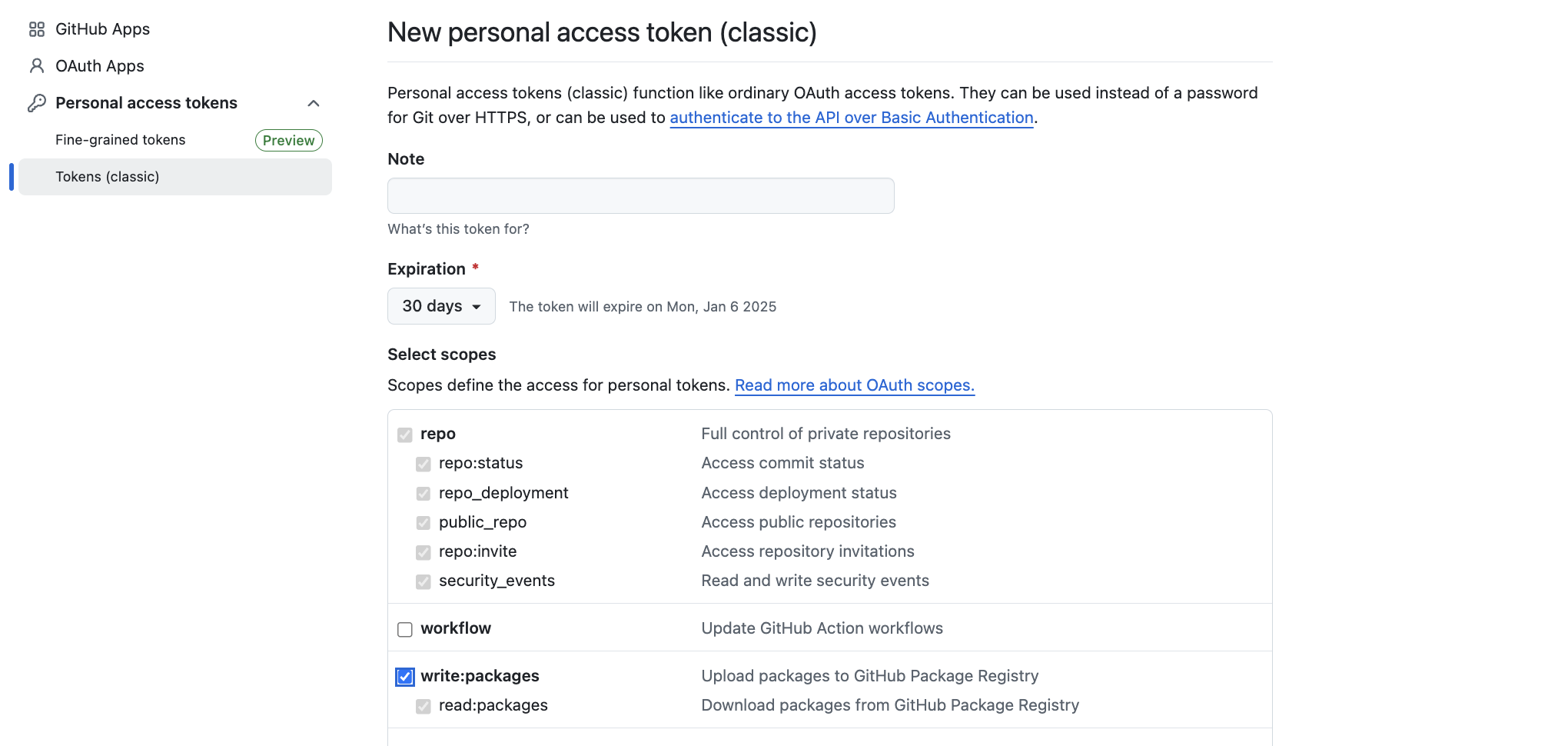
npm login --registry=https://npm.pkg.github.com
# Username: Your GitHub username
# Password: Paste your Personal Access Token
# Email: Your GitHub email
Build and publish
# Build the package
npm run build
# Publish to GitHub Packages
npm publish
Installing the packages
Create a .npmrc file in your project directory and add this @your-github-username:registry=https://npm.pkg.github.com/
pnpm install @your-github-username/my-typescript-library